Swift iOS to Raspberry Pi TV Remote Control
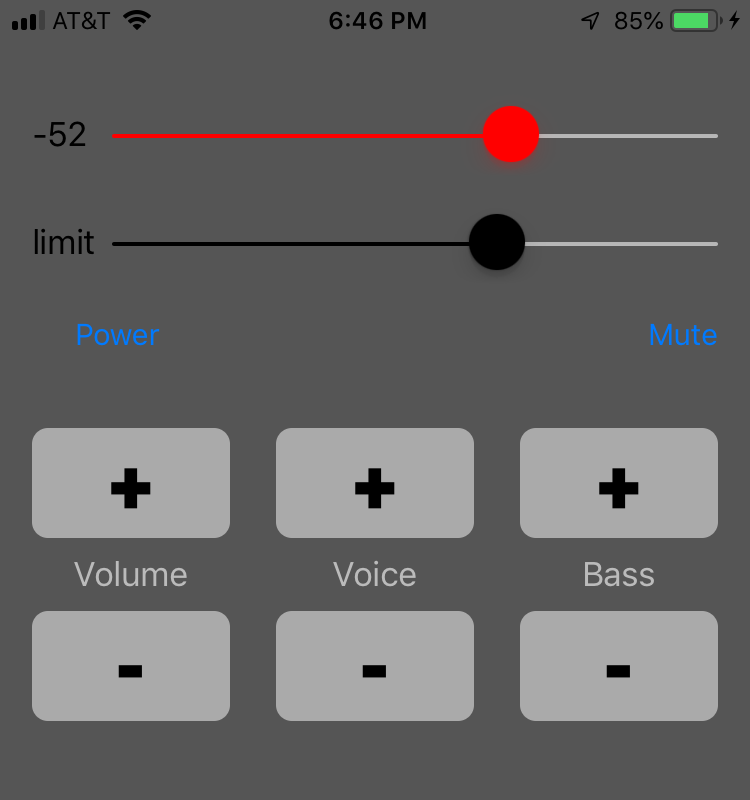
I made an iOS app to remote control a television sound bar.
The app makes requests to a raspberry pi running a Flask server on the local network. The Raspberry Pi uses LIRC (Linux Infrared Remote Control) to send commands to an attached infrared transmitter.
Audio level
AudioMonitor.swift levelTimerCallback repeatedly posts a notification containing audio level. AVAudioRecorder averagePower(forChannel:) normally ranges from -160 dB (quietest) to 0 dB full scale (louder). “If the signal provided to the audio recorder exceeds ±full scale, then the return value may exceed 0 (that is, it may enter the positive range).” Indoors I typically see range approximately -60 to -30 dB.
@objc func levelTimerCallback() {
guard let recorder = recorder else { return }
// To obtain a current average power value,
// you must call the updateMeters() method before calling averagePower.
recorder.updateMeters()
let level = recorder.averagePower(forChannel: 0)
let isLoud = level > levelDbThreshold
print("levelTimerCallback sound level: \(level) decibel, isLoud: \(isLoud)")
DispatchQueue.main.async {
NotificationCenter.default.post(name: AudioMonitor.audioLevelNotificationName,
object: .none,
userInfo: [AudioMonitor.audioLevelKey: level,
AudioMonitor.isLoudKey: isLoud])
}
}
Automatic control
The iOS app could be modified to automatically send a volume decrease command whenever the audio level exceeds the limit.
Alternatively, the Raspberry Pi app remy_python could be modified to automatically control sound level. For example the Pi could automatically decrease or mute the sound whenever a commercial is detected e.g.
- at regularly scheduled commercial times (e.g. 19:02, 19:30)
- analyzing closed captioning text for a word on a list (e.g. “Lexus”, “Tylenol”)
References
Remy
https://github.com/beepscore/Remy
Remote control television by sending commands from iOS device to a server.
iOS - Detect Blow into Mic and convert the results! (swift)
https://stackoverflow.com/questions/31230854/ios-detect-blow-into-mic-and-convert-the-results-swift
how to monitor audio input on ios using swift - example?
https://stackoverflow.com/questions/35929989/how-to-monitor-audio-input-on-ios-using-swift-example
Network enabled Raspberry Pi tv remote control
https://beepscore.com/website/2019/02/05/network-enabled-raspberry-pi-tv-remote-control.html
Raspberry Pi infrared remote control for a television sound bar
https://beepscore.com/website/2019/02/03/raspberry-pi-infrared-remote-control-tv.html
remy_python
https://github.com/beepscore/remy_python
Make a Raspberry Pi infrared remote control. The device can programmatically control television sound bar audio volume. The Raspberry Pi uses LIRC (Linux Infrared Remote Control) to send commands to an attached infrared transmitter.
The Python app has two parts:
- Functions to send commands to the infrared transmitter, which then transmits the commands to the television sound bar
- A Flask web service to accept television command requests (e.g. volume decrease, volume increase).
The README includes links to similar remote control projects, infrared remote control hardware.